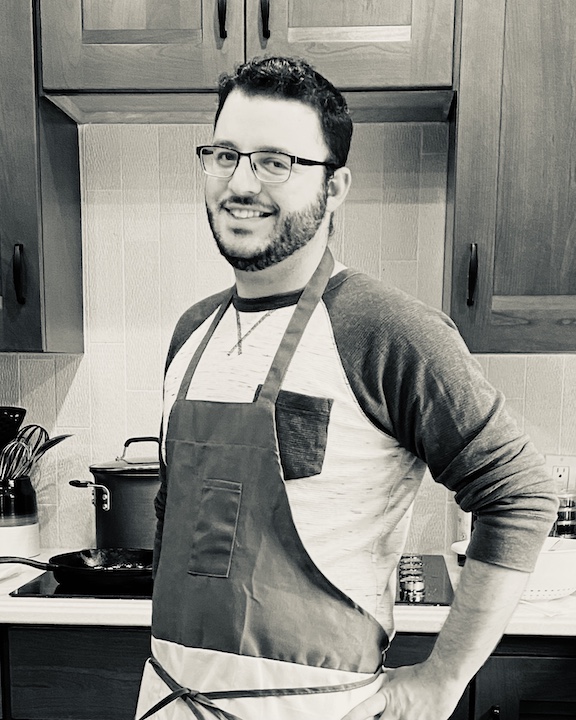
Welcome!
I am a software engineer with a passion for problem solving.
With over a decade of experience as a software engineer, my technical expertise focuses on software architecture, disciplined engineering practices, and strategic leadership and technical management to create solutions that solve impactful problems.
Aside from my professional interests, I enjoy a variety of intellectual curiosities from crossword puzzles and astronomy to guitar and cooking.